How To Compare Two Strings In Dev C++
Stack Overflow Public questions and answers; Teams Private questions and answers for your team; Enterprise Private self-hosted questions and answers for your enterprise; Talent Hire technical talent. How to find a substring inside a string in C using the find standard library function. Advantage of C Standard Library String functions that are most commonly used by C developers for their day to day development operations. The C Standard Library contain a lot of useful information when working with string functions and before. C Program to Compare Two Strings. To compare any two string first we need to find length of each string and then compare both strings. If both string have same length then compare character by character of each string. To understand below example, you have must knowledge of following C programming topics; For Loop in C, While Loop in C.
I am trying to learn C++ and am trying to figure out how to use strings and if statements together. Here's the code I'm trying to play around with:
Comparing String objects using Relational Operators in C Search in an array of strings where non-empty strings are sorted Meta Strings (Check if two strings can become same after a swap in one string). Std::string::compare in C compare is a public member function of string class. It compares the value of the string object (or a substring) to the sequence of characters specified by its arguments. Compare Two Strings in C. To compare two string in C Programming, you have to ask to the user to enter the two string and start comparing using the function strcmp.If it will return 0, then both string will be equal and if it will not return 0, then both string will not be equal to each other as shown in here in the following program.

Every time I type in no, the statement 'You open the door' pops up. Anybody know what I'm doing wrong?
Thanks
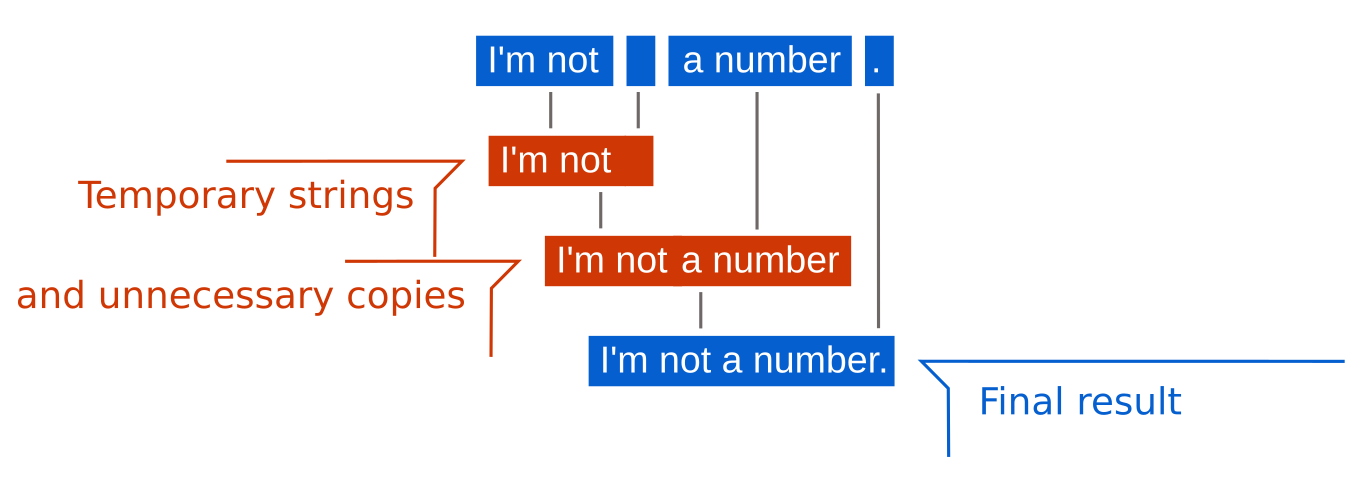
- 5 Contributors
- forum 5 Replies
- 4,946 Views
- 1 Year Discussion Span
- commentLatest Postby sftrannaLatest Post
mike_2000_172,669
First of all, for any type (string or other), the statement 'if ( a = b )' does not check whether a is equal to b, it assigns the value of b to a, and returns the final value a (which is also the value of b). The single = sign is an assignment operator, not a comparison operator. The comparison for equality is , i.e. double equal sign.
Second, the strings that you are using are so-called C-strings (kept for legacy support of C code in C++). The proper string to use is the class 'std::string' (in the '#include <string>' header. Using these, your code will work (with the double equal sign instead of single equal sign for comparisons).
Comparing Strings In C
Third, if you have to use 'char *', i.e. C-strings, then there is no equality operator for it, so 'a 'yes' will not work. The proper function to compare two C-strings is strcmp(), which will return 0 if they are equal. Thus:
Compare Two Strings Online
Finally, and most importantly, having 'char* a;' does not initialize 'a'. 'a' is a pointer to an array of characters (char). By not initializing it, you have a pointer that points nowhere (well it points somewhere, but not somewhere that it should point to, because the memory at that address will be used for something else and using 'a' uninitialized will corrupt your program). So, you need to initialize it by giving some space for it. Since you are beginning to learn, I'm not sure how much I should or could explain, so I will just say that you should replace line 5 by this:
How To Compare Two Strings In Dev C Pdf
But, frankly, using std::string is highly recommended here.